My first module - Admin Settings
So I'm not great at PHP. I don't really understand Drupal hooks all that well, but I have been wanting to work on a module for a while now. So I took on the project of making a module that connects drupal with TeamworkPM, which is our task management system we use.
My files are teamwork.info, teamwork.module, teamwork.rules.inc, and teamwork.install. I am going to show how I set up the admin settings in this blog
Admin Settings Form
Originally i was using drupal's admin form system_settings_form for the settings, whcih was easy to set up, and used set_variable for the API key and the Company name which I can than grab using get_variable. I had to change this later though, because I wanted each user to be able to enter their own API key and Company name. So I had to add a table in the database, then populate that table with the form values using db_insert. I can then grab those values using db_query. Here is my code:
.install file
function teamwork_schema() {
$schema['teamwork_api'] = array(
'description' => 'The API key',
'fields' => array(
'api_key' => array(
'description' => 'Teamwork API key',
'type' => 'varchar',
'length' => 256,
'not null' => TRUE,
),
'company_name' => array(
'description' => 'The Company Name',
'type' => 'varchar',
'length' => 256,
'not null' => TRUE,
),
'uid' => array(
'description' => 'The unique user id',
'type' => 'int',
'length' => 3,
'not null' => TRUE,
'default' => 1,
),
),
);
return $schema;
}
.module file
function teamwork_admin_form ($form, &$form_state) {
//$teamwork_schema = drupal_get_schema('teamwork_api');
global $user;
$api_key = db_query('SELECT api_key FROM {teamwork_api} WHERE uid = :uid', array(':uid' => $user->uid))->fetchField();
$company_name = db_query('SELECT company_name FROM {teamwork_api} WHERE uid = :uid', array(':uid' => $user->uid))->fetchField();
$form = array();
$form['teamwork_settings_markup'] = array(
'#type' => 'markup',
'#markup' => '<div>
<h3>You need to get your API key from teamwork in order for this module to work</h3>
<p>You can go to http:// developer.teamwork.com/enabletheapiandgetyourkey for more information
</div>
',
);
$form['teamwork_key'] = array(
'#type' => 'textfield',
'#title' => t('Please enter your Team Work API Key') ,
'#description' => t("The maximum number of links to display in the block.") ,
'#default_value' => $api_key,
'#required' => FALSE,
);
$form['company_name'] = array(
'#type' => 'textfield',
'#title' => t('Please enter your Company Name') ,
'#description' => t("The maximum number of links to display in the block.") ,
'#default_value' => $company_name,
'#required' => FALSE,
);
$form['teamwork_admin_form_submit'] = array(
'#type' => 'submit',
'#value' => t('Submit'),
);
return $form;
}
function teamwork_admin_form_submit($form, &$form_state) {
global $user;
$api_key = db_query('SELECT api_key FROM {teamwork_api} WHERE uid = :uid', array(':uid' => $user->uid))->fetchField();
$company_name = db_query('SELECT company_name FROM {teamwork_api} WHERE uid = :uid', array(':uid' => $user->uid))->fetchField();
if(empty($api_key)) {
drupal_set_message('Your API key and company name have been successfully added.');
$result = db_insert('teamwork_api') // Table name no longer needs {}
->fields(array(
'api_key' => $form_state['values']['teamwork_key'],
'company_name' => $form_state['values']['company_name'],
'uid' => $user->uid,
))
->execute();
return $result;
}
else {
drupal_set_message('Your API key and company name have been successfully updated.');
$result = db_update('teamwork_api') // Table name no longer needs {}
->fields(array(
'api_key' => $form_state['values']['teamwork_key'],
'company_name' => $form_state['values']['company_name'],
'uid' => $user->uid,
))
->execute();
return $result;
}
}
About the Author:
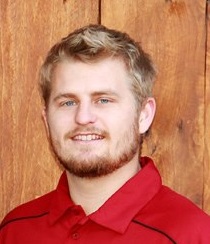
Tommy Sliker
Web Developer
Tommy Sliker, aka Mirakolous, is a creative mastermind, Drupal Front End guru, and a contributor to Drupal Core. He has been producing his own music since he was twelve years old, and has a wide breadth of creative skills including photography, video production, and graphic design.
In addition to his Web work, Tommy's passions include music production, drums, guitar, photography,